Advanced E-mail Notifications
This form will ask the user "What can we help you with?", and send a notification to a different e-mail address depending on the answer selected.
This tutorial will extend on the tutorial that covers basic contact enquiry forms, to show how server code can be used to change the form notification email address based on fields selected by the user.
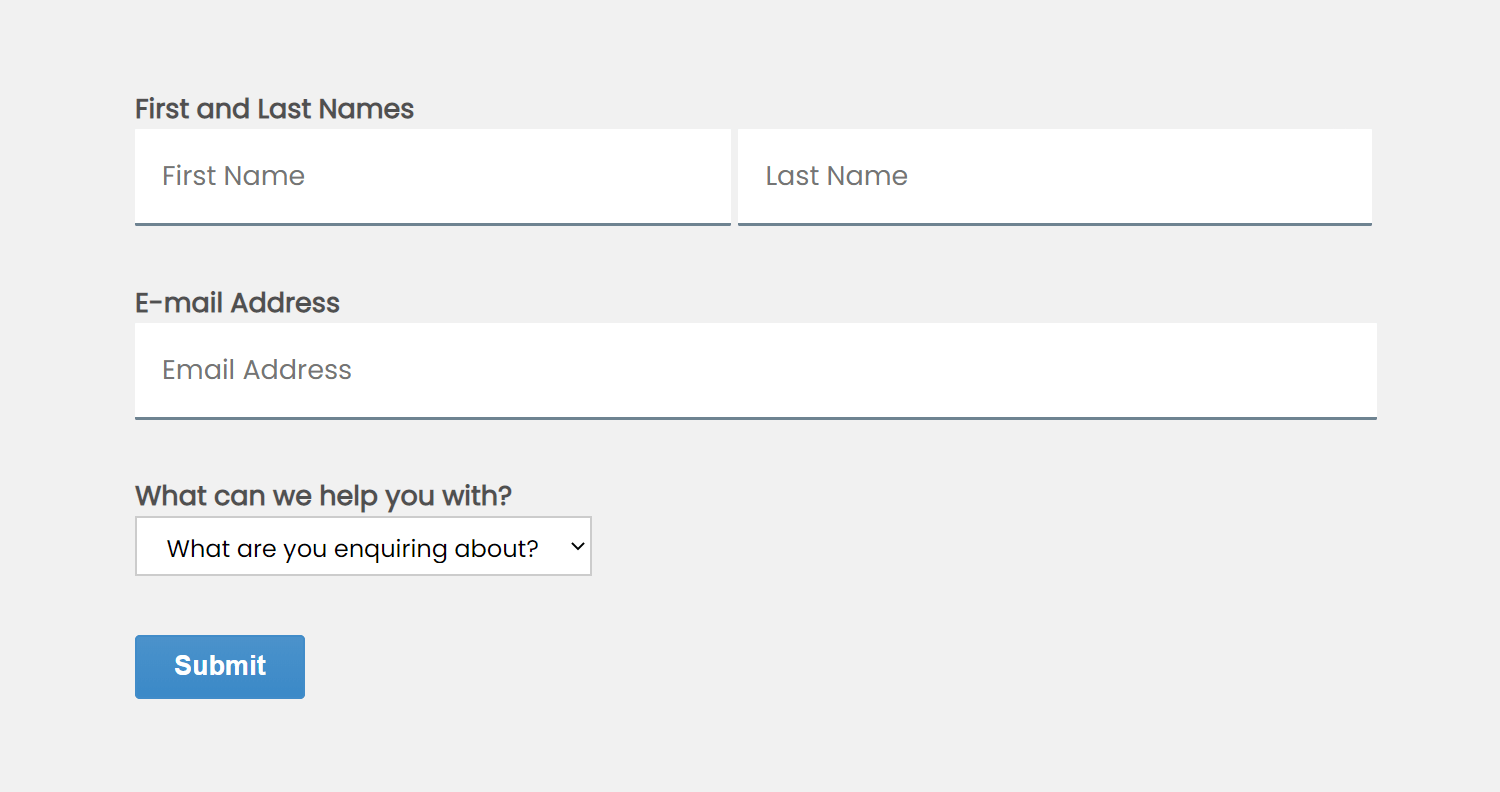
The Form
To get started, add the following form code to a page:
Combobox
The <forms:combobox> control on line 10 allows the user to select between a range of options for "What can we help you with?". Click here to read more about the combobox control.
The combobox can be referenced by its ID:
$form_reason.getValue()
Passing Fields to Server Code
Rather than providing a specific notification e-mail address in the onsubmitemail attribute of the form, a custom function will be used to return an e-mail address based on the value of the combobox.
In this case we only need the value of a single combobox, however where you need to send more, or all field values to a function, you can use $my_form_id.getValues() to retrieve all form field values (read more here).
The Server Code
Custom PHP functions can be added via the PHP tab, which is located in the source view when editing a page. Where PHP functions need to be used across multiple pages, the function should be added to the website design instead.
Add the following PHP code to the page (edit the page, open src view, click the PHP tab, and paste):