User Interface Controls & PHP API
Oncord features a library of User Interface Controls for adding dynamic elements to your pages, such as web forms, galleries and navigation menus.
The Oncord PHP API provides a library of methods that can be used to retrieve or manipulate data from Oncord (for example, data associated with Contacts, Pages, Posts or Products).
User Interface Controls
Oncord User Interface controls are an extension of the standard HTML element library, and use the same "tags" syntax.
Where HTML elements use attributes to provide additional information (such as class, id etc), Oncord UI Controls also have a set of attributes to control how elements behave and display data.
Standard HTML attributes such as width, height, class and id can be used with UI Controls.
To view a full list of UI Controls and all their available attributes, visit the Controls API Reference.
Example: Images
As an example, you can opt to use standard html <img> tags with Oncord (or any other standard HTML tag). However, the <standard:image> UI Control offers some improvements, such as image cropping and thumbnail generation.
The attribute "maintainaspect" in the above example determines how the image should respond if the width and height set are a
different ratio to the image's natural size.
Example: Page Sections
You've likely encountered the <section> HTML element, which is used to group content into "sections".
In order to provide users with more flexibility editing website pages, Oncord provides a similar <templates:section> UI control.
This element can be dragged into position using the visual page editor, similarly to many other common Oncord UI controls.
Alternatively, you can manipulate source code directly, by clicking the SRC button while editing pages or designs.
When selected via Oncord's visual page editor, some attributes of this control are shown as options in the left panel, providing users
with control over the element without the need to manipulate source code directly.
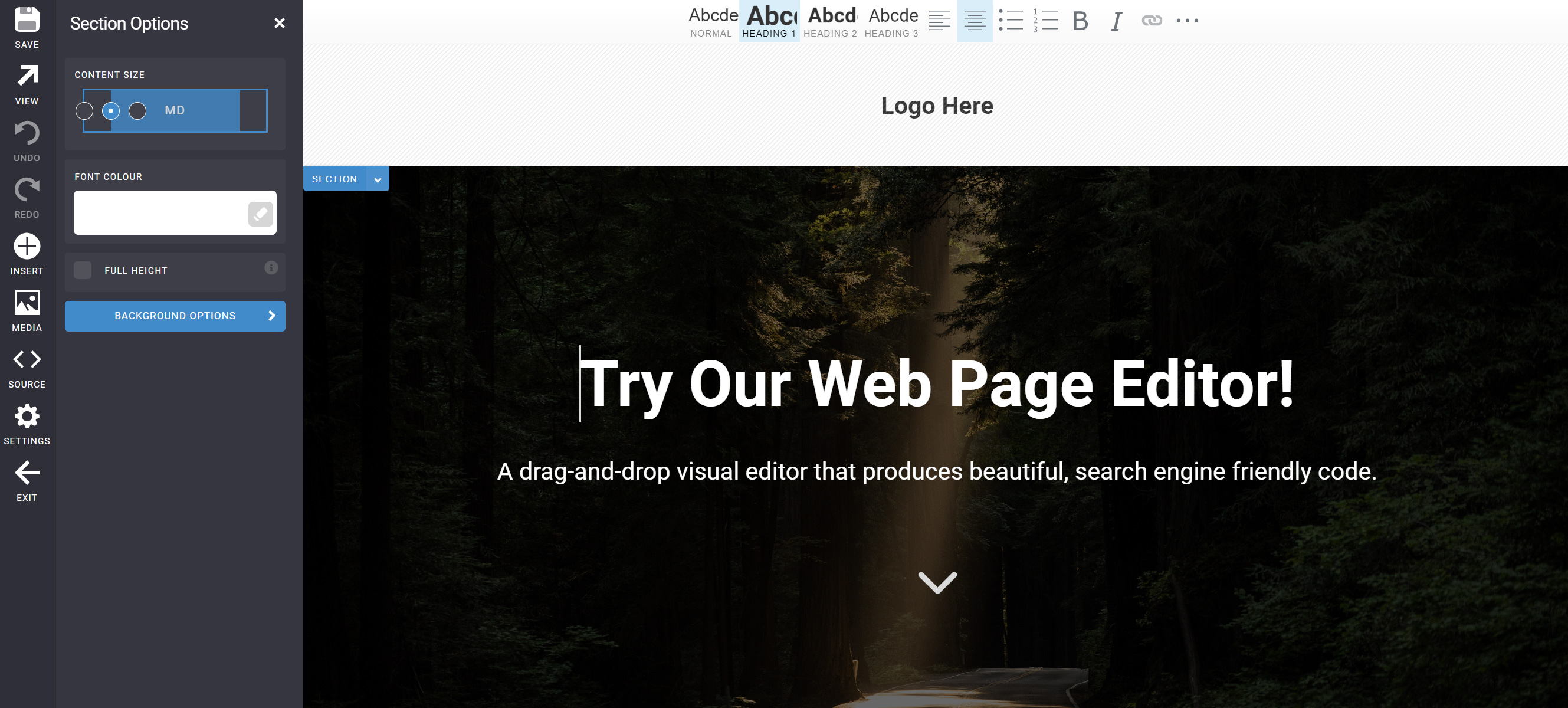
- Change the background of the section to an image, solid colour, or gradient.
- Control the container size of the section, or make this section "full height".
- Define an inline font colour.
Example: Website Navigation
When administrators create, re-name or delete website pages, those changes usually need to be reflected in the website navigation menu.
A "Primary Navigation" UI Control can be used to dynamically populate the menu based on the Page structure of the website.
Rather than coding a static HTML list of links like this example:
A Primary Navigation UI Control can be used to produce the above list dynamically:
The UI Control's attributes are used to control which pages should be included in the nav menu, and whether some default CSS styling should
be applied.
The Oncord PHP API
PHP is a widely used server-side scripting language that can be embedded into HTML to build web applications and dynamic websites.
The Oncord PHP API provides a library of methods that can be used to retrieve or manipulate data from Oncord (for example, data associated with Contacts, Pages, Posts or Products).
Oncord's PHP API is logically sorted by Components:
\Components\Website\Pages::currentPageId()
The <logic:variable> UI control offers a good example to illustrate how to retrieve and display data throughout website pages. This UI control sets a new variable to be used throughout the document.
The "as" attribute defines the name of the variable, and the "value" attribute defines a data source (usually an API call to retrieve a Page, Product, Contact etc).
Try This Now: Edit a website page, click the SRC button, and paste the following code:
The above example creates a new variable $page. The value is set to an API call, which will return an array of fields describing the home
page (title, SEO description, whether the page is hidden in navigation etc).
To change which page is retrieved, change the page address defined in the value attribute (ie. change '/' to '/about-us/').
You'll notice this UI control doesn't render any data on the page, it only sets a variable for use in your document.
Some additional code is needed to render the variable on the page (more below).
Templating Syntax
Oncord uses a simple templating syntax to add dynamic content to a page.
An expression can be placed anywhere in the document, starting with [? and ending with ?]
You can use this approach to render the fields from the $page variable set in the example above:
Important:
This syntax is used when displaying data on the page, as well as inside the value attribute, in order to parse the expression.
[? expression ?] Single question mark notation html encodes any string output from the expression, meaning that html code in your output will be displayed as text.
[?? expression ??] Double question mark syntax leaves output unencoded.
In the example above, the 'page_content' field of a page contains html content. By using the double question mark notation, we can
render the html content, rather than display it as text.
Fields & API Methods
The above example can be easily modified to display a Post, instead of a Page:
As mentioned above - The PHP API is logically sorted by Oncord Components:
\Components\Website\Posts
Click here to view the PHP API reference for Posts.
In the API reference for Posts, you'll find a list of methods that can be used to retrieve or manipulate data - examples such as:
- get() which will retrieve a specific Post.
- getAll() which will retrieve all Posts.
- getAllForCategory() which will retrieve all Posts of a specific Post Category.
Below that list of methods, you'll find a list of relevant database fields.
In the list of fields associated with Posts, you'll find "post_content_short", which is the Post Short Description field.
Here's how the above example could be modified to add the Post Short Description field:
The function varDump($variable) can also be used to render all data associated with a variable, which can be useful troubleshooting issues: